PHP Web Development: Lesson Three - Mastering Sessions, Cookies, and File Uploads
In this third installment of our PHP web development series, we delve into advanced features that enhance user experiences and streamline data management. This lesson covers the implementation and management of sessions and cookies, crucial for maintaining user data and preferences across multiple page accesses. Additionally, we introduce file uploads, allowing users to transfer files to your server seamlessly. Through practical examples and detailed explanations, readers will learn how to effectively utilize these tools in their PHP projects, paving the way for more interactive and personalized web applications.
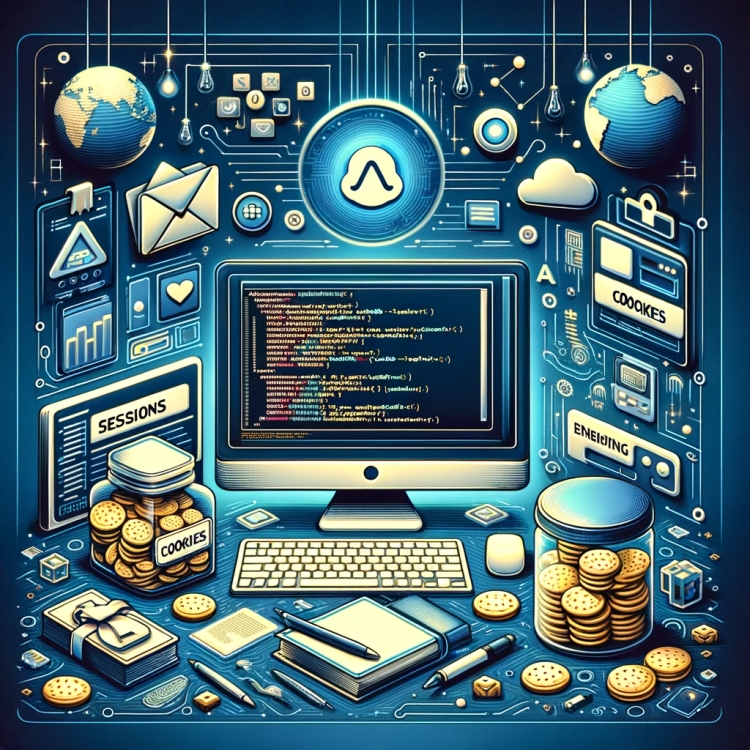
After covering the basics of PHP, form handling, interacting with databases, and securing your applications, it's time to delve into features that make web applications more dynamic and user-friendly. In this lesson, we focus on sessions and cookies for maintaining state and user data across pages, and we introduce handling file uploads—a common requirement for many web applications.
1. Understanding and Managing Sessions in PHP
Sessions provide a way to preserve certain data across subsequent accesses. This functionality is essential for identifying users across different pages of your application.
1.1 Starting a Session
To use sessions in PHP, start by calling session_start()
at the beginning of your script:
<?php
session_start();
?>
This function must be called before any output is sent to the browser.
1.2 Storing and Accessing Session Data
You can store data in the $_SESSION
superglobal array. For example, to save a username:
<?php
$_SESSION['username'] = 'JohnDoe';
?>
Access this data on subsequent pages by starting the session and then referencing the $_SESSION
array:
<?php
session_start();
echo 'Hello, ' . $_SESSION['username'];
?>
2. Using Cookies to Remember User Preferences
Cookies allow your application to store data on the user's computer. They are commonly used for remembering login details and user preferences.
2.1 Setting Cookies in PHP
Set a cookie using the setcookie()
function. Here’s how to create a cookie that remembers a user's name:
<?php
setcookie("user", "John Doe", time() + (86400 * 30), "/"); // 86400 = 1 day
?>
2.2 Accessing Cookies
Access cookies via the $_COOKIE
superglobal array. For example, to display the user's name stored in a cookie:
<?php
if(!isset($_COOKIE["user"])) {
echo "Guest";
} else {
echo "Hello, " . $_COOKIE["user"];
}
?>
3. Handling File Uploads
File uploads enable users to send files from their computer to your server, an essential feature for many applications.
3.1 Creating a File Upload Form
Start with an HTML form that specifies an encoding type of multipart/form-data
:
<form action="upload.php" method="post" enctype="multipart/form-data">
Select image to upload:
<input type="file" name="fileToUpload" id="fileToUpload">
<input type="submit" value="Upload Image" name="submit">
</form>
3.2 Processing the Uploaded File in PHP
In your PHP script, you can access the uploaded file through the $_FILES
superglobal array. Here's how to save the uploaded file to a specific directory:
<?php
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileToUpload"]["name"]);
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)) {
echo "The file ". htmlspecialchars( basename( $_FILES["fileToUpload"]["name"])). " has been uploaded.";
} else {
echo "Sorry, there was an error uploading your file.";
}
?>
What's Your Reaction?
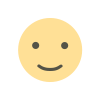
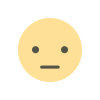
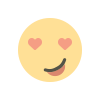
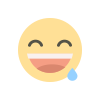
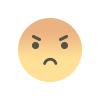
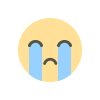
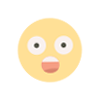