PHP Web Development: Lesson Two - Working with Form Data, Databases, and Security
This blog post delves into the foundational aspects of web development using PHP, focusing on three critical areas: handling form data, database interaction, and implementing essential security measures. Starting with a guide on creating and processing HTML forms, it then explores establishing secure connections and operations with databases via PHP Data Objects (PDO). The final section addresses crucial security practices to safeguard your web applications against common threats. Through concise explanations and practical examples, this lesson aims to equip you with the necessary skills to enhance user interaction, data management, and security in your PHP projects.
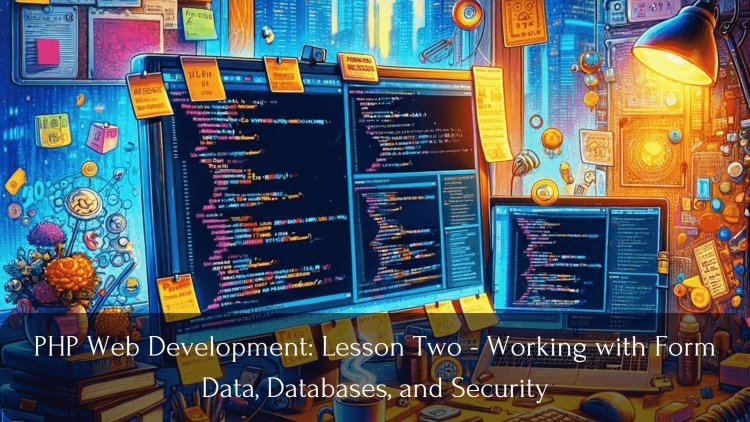
At the heart of modern web applications lie user interaction, data storage, and security. PHP offers robust tools to work with these fundamental components. In our second lesson, we delve deeper into these essential aspects of PHP.
1. Working with Form Data: Collecting Information from Users
1.1. Creating HTML Forms
One of the most common methods of user interaction in web applications is through forms. PHP can easily process data sent via HTML forms. Let’s start with a simple form example:
<!-- A Simple HTML Form -->
<form action="process_form.php" method="post">
Your Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
1.2. Processing Form Data with PHP
We use PHP's superglobals to process the submitted form data. Access and process form data using the $_POST
superglobal:
<?php
// process_form.php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = htmlspecialchars($_POST['name']);
echo "Hello, " . $name;
}
?>
2. Interacting with Databases: Storing and Querying Data
An important aspect of web applications is interaction with databases. PHP can interact with popular databases like MySQL, providing ways to store and retrieve data efficiently.
2.1. Establishing a Database Connection with PDO
PHP Data Objects (PDO) offers a secure way to connect to a database. Here’s how you can establish a connection to a MySQL database:
<?php
try {
$pdo = new PDO("mysql:host=localhost;dbname=database_name", "username", "password");
// Set the PDO error mode to exception
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch(PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
2.2. Inserting Data into the Database
Securely insert data into the database using PDO’s prepare
and execute
methods:
<?php
$stmt = $pdo->prepare("INSERT INTO users (name) VALUES (:name)");
$stmt->bindParam(':name', $name);
$name = "John";
$stmt->execute();
echo "New record created successfully.";
?>
This example demonstrates how to securely insert data into the database.
3. Security Measures: Protecting Your Application
Securing your web application is crucial to maintaining the trust of your users. Protect against common attacks such as SQL injection, XSS, and CSRF by implementing security measures.
3.1. Sanitizing and Validating User Input
Always sanitize and validate user inputs to prevent security vulnerabilities. Functions like htmlspecialchars
and filter_var
can help in this process.
3.2. Preventing SQL Injection
Use PDO’s prepare
and execute
methods to securely execute SQL queries. These methods provide effective protection against SQL injection attacks.
What's Your Reaction?
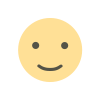
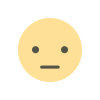
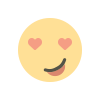
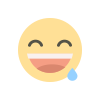
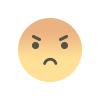
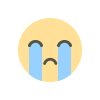
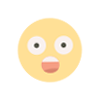