PHP Coding Lesson: Introduction to Server-Side Programming, Basic PHP Syntax, and Working with Variables
Embark on your journey into the world of server-side programming with our "PHP Coding Lesson." This session is designed for beginners and aims to lay the groundwork for developing dynamic web pages using PHP. We'll start with a comprehensive introduction to PHP, explaining its significance in the web development landscape. Following that, we'll dive into the essentials of PHP syntax, guiding you through the process of writing your very first PHP scripts. You'll learn about declaring variables, manipulating data, and the basics of data types in PHP. By the end of this lesson, you'll have a clear understanding of PHP's fundamental concepts, setting the stage for more advanced topics in subsequent lessons. Whether you're aspiring to become a web developer or simply looking to expand your programming skills, this lesson will provide you with the knowledge and tools to start coding in PHP.
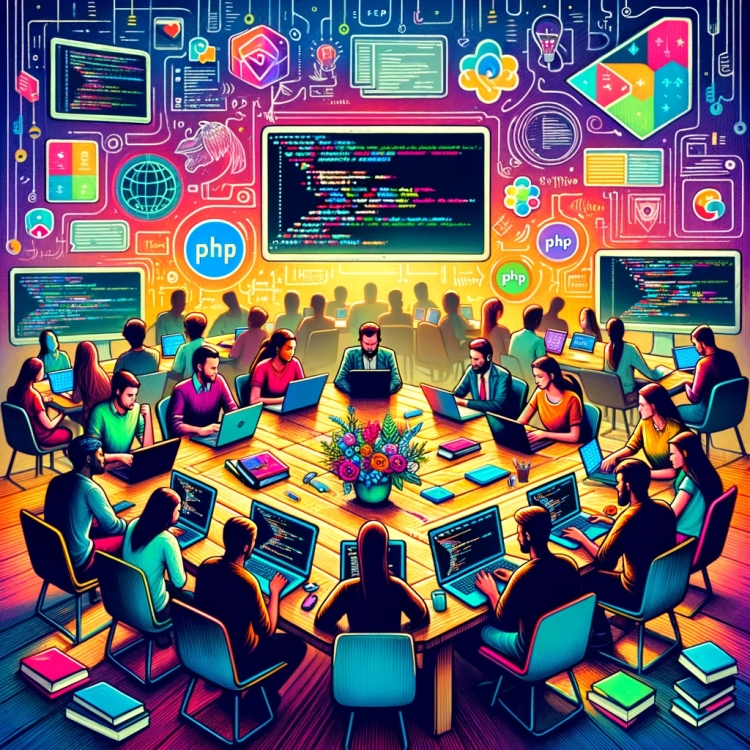
Starting with PHP is a significant first step in web development. PHP, or Hypertext Preprocessor, is a widely used server-side scripting language that's especially valuable for creating dynamic web pages and applications. If you're embarking on learning PHP, this series is designed to guide you from the basics to more advanced concepts. Let's dive into the first lesson.
PHP Series: Lesson 1 - Introduction to PHP
What is PHP?
PHP is a server-side scripting language that allows web developers to add dynamic content to websites. It can interact with databases, handle form data, and perform many other web-related tasks.
Setting Up Your Environment
To start writing and testing PHP scripts, you need to set up a local server environment on your computer. Tools like XAMPP, WAMP, or MAMP can help you create this environment by packaging PHP, MySQL, and Apache Server together.
- XAMPP/WAMP/MAMP: Choose one to install on your computer. These tools will provide you with the necessary server environment to run PHP scripts.
- Editor: You will also need a text editor or an Integrated Development Environment (IDE) to write your code. Recommended options include Visual Studio Code, Sublime Text, or PhpStorm.
Basic Concepts and Examples
1. PHP Syntax
PHP code is written between <?php
and ?>
tags. This tells the PHP engine to interpret the enclosed code as PHP.
<?php
echo "Hello, PHP!";
?>
2. Variables
Variables in PHP start with a $
sign and are used to store data.
<?php
$message = "Hello, PHP!";
echo $message;
?>
3. Conditional Statements
Use if
and else
statements in PHP to perform actions based on conditions.
<?php
$number = 4;
if ($number > 5) {
echo "Number is greater than 5.";
} else {
echo "Number is 5 or less.";
}
?>
4. Loops
PHP supports loops like for
, while
, and foreach
to execute code blocks multiple times.
<?php
for ($i = 1; $i <= 5; $i++) {
echo $i . " ";
}
?>
5. Functions
Functions in PHP are blocks of code designed to perform a particular task, defined by the function
keyword.
<?php
function sayHello() {
echo "Hello, PHP!";
}
sayHello();
?>
Conclusion and Next Steps
This lesson introduced you to PHP and covered the basics needed to start developing dynamic web pages. You learned about PHP syntax, variables, conditional statements, loops, and functions. In the next lesson, we will delve into more advanced topics like working with forms, handling user input, and basic database operations.
Stay tuned for more lessons in this series, designed to build your PHP skills progressively. Happy coding!
What's Your Reaction?
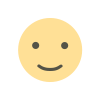
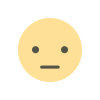
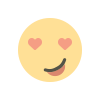
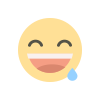
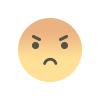
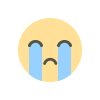
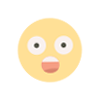